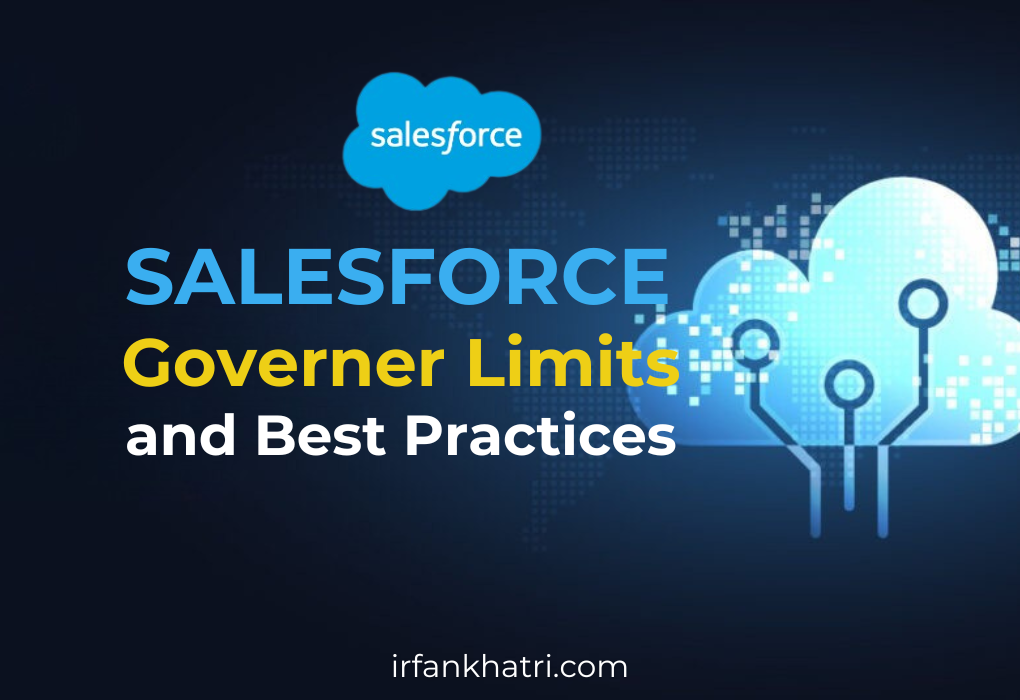
Salesforce Governor Limits and Best Practices
Salesforce is a powerful platform for building and deploying cloud-based applications. However, one of the unique aspects of Salesforce is its multi-tenant architecture, which shares resources among multiple customers.
This architecture requires strict management of resource usage to ensure fair access and optimal performance for all customers. Governor limits are Salesforce’s way of enforcing resource usage limits, ensuring that a single user’s or organization’s code does not consume excessive resources or degrade the platform’s performance for others.
This article will delve into Salesforce’s governor limits, including the types of limits, common errors, and best practices for optimizing your code to avoid them.
What Is Salesforce Multitenancy?
Salesforce operates on a multi-tenant architecture. This means multiple customers share the same infrastructure (servers, databases, etc.) but have isolated, secure environments for their data and applications. This allows Salesforce to serve thousands of businesses on a single platform while maintaining data privacy, security, and performance.
In a multi-tenant environment, resources are shared. As a result, heavy consumption by one tenant can negatively affect the performance of others. To mitigate this, Salesforce has implemented governor limits. These limits ensure fair usage of resources across all customers.
What Are Salesforce Governor Limits?
Governor limits are a set of restrictions Salesforce imposes to control the consumption of system resources. These limits apply to various aspects of the platform, such as database queries, DML operations, and CPU time. The main goal is to prevent one piece of code from monopolizing resources and ensure a balanced experience for all users.
These limits are enforced to protect the shared infrastructure. They help ensure the platform remains scalable and reliable. If a piece of code exceeds a governor limit, Salesforce throws an error, typically preventing the transaction from completing.
Types of Salesforce Governor Limits
Salesforce imposes various governor limits on developers to ensure efficient resource usage. Some common types include:
1. Per-Transaction Apex Limits
These limits count for each Apex transaction. For Batch Apex, these limits are reset for each execution of a batch of records in the execute method.
2. Per-Transaction Certified Managed Package Limits
Per-Transaction Certified Managed Package Limits apply to Apex code within managed packages that have been certified by Salesforce. These limits are slightly higher than those for normal Apex transactions but still ensure that the managed package operates within the platform’s resource constraints to avoid performance degradation in a multi-tenant system.
3. Lightning Platform Apex Limits
These limits aren’t specific to an Apex Transaction and are enforced by the Lightning platform. They ensure that the platform performs optimally while allowing developers to build and deploy custom logic within specified resource constraints.
4. Static Apex Limits
These limits apply across all transactions, for example, restricting loop and Apex trigger sizes, callout sizes and times, trigger code unit numbers, etc.
5. Size-Specific Apex Limits
These limits are related to the size of code, for example, maximum number of characters for a class or trigger.
6. Push Notification Limits
These limits apply to the number of push notifications that can be sent to mobile devices or other platforms within a specified time period.
There are also other types of governor limits. They’re called non-Apex limits:
- Callout Limits
- General Email Limits
- Chatter Limits
- Metadata Limits
- API Request Limits and Allocations
- Connect REST API Limits
- API Query Cursor Limits
- SOAP API Call Limits
- SOQL and SOSL Limits for Search Queries
- Visualforce Limits
How to Bypass Salesforce Governor Limits
Knowing the limits is important, but applying best practices to manage them effectively is crucial. Here are some best practices to keep in mind when developing on Salesforce:
1. SOQL Limit (Too many SOQL queries: 101)
Avoid querying inside loops. Always try to fetch records in bulk, ideally outside of loops, to minimize the number of queries.
Bad Practice:
for (Account acc : [SELECT Id FROM Account WHERE Name LIKE 'Test%']) {
List<Contact> contacts = [SELECT Id FROM Contact WHERE AccountId = :acc.Id];
// Perform operations with the contacts...
}
Good Practice:
List<Account> accounts = [SELECT Id, (SELECT Id FROM Contacts) FROM Account WHERE Name LIKE 'Test%'];
for (Account acc : accounts) {
for (Contact con : acc.Contacts) {
// Perform operations with the contacts...
}
}
2. Limit DML Statements (Too Many DML Statements: 151)
Combine multiple DML operations to process records in bulk rather than executing a DML operation for each record individually.
Bad Practice:
for (Account acc : [SELECT Id FROM Account WHERE Name LIKE 'Test%']) {
acc.Name = 'Updated Name';
update acc; // DML operation inside loop
}
Good Practice:
List<Account> accountsToUpdate = new List<Account>();
for (Account acc : [SELECT Id FROM Account WHERE Name LIKE 'Acme%']) {
acc.Name = 'Updated Name';
accountsToUpdate.add(acc);
}
// Perform bulk DML operation outside the loop
update accountsToUpdate;
3. Limit Rows (Too Many Query Rows: 50001)
Running a single SOQL query that retrieves an excessively large number of records without any limit and filters. To solve this issue, limit and filter in your SOQL query or use Batch Apex to break the data into smaller chunks.
Bad Practice:
// This may return more than 50,000 records, exceeding the limit.
List<Account> accounts = [SELECT Id, Name FROM Account];
Good Practice:
// Using a filter to limit the number of records
List<Account> accounts = [SELECT Id, Name FROM Account WHERE CreatedDate > :startDate LIMIT 50000];
Summary
Salesforce governor limits are a critical aspect of developing efficient and scalable applications on the Salesforce platform. By following best practices like avoid querying inside loops, combine multiple DML operations, and limit and filter in your SOQL query, you can ensure that your code runs smoothly while staying within these limits.